Week 2 - Iceberg Simulation
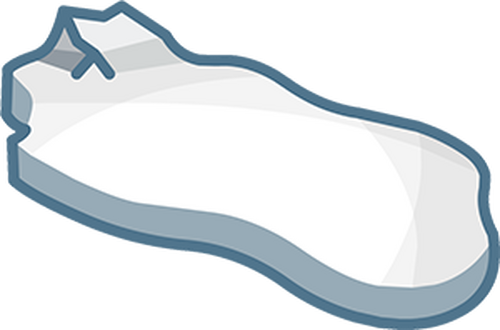
Week 2 - Simulate an Iceberg in p5.js
Step 1 - REVIEW
I'm starting off this assignment by using the random perlin noise and random walk sketches we worked on in class as references for my slow moving iceberg. They are simple and easy to follow, however, I currently have no idea how I am going to approach combining the drawing of our iceberg's position with both random perlin noise coordinates AND our random x and y walk coords. Here's what the perlin noise code I'm focusing on right now looks like in contrast to the random walk code:
Perlin Noise: Random Walk:
```
let x = 300 * noise(frameCount * 0.002) x+=random(-2,2);
let y = 400 * noise(frameCount * 0.003) y+=random(-3,2);
```
Step 2 - THINK
Uhhh...
My first approach is to open up a sandbox sketch and try my hand at combining the code for our x and y variables. Here's what I am going to try first:
```
let x = 300 * noise(frameCount * 0.002)
let y = 500 * noise(frameCount * -0.006)
y += random(-3,1);
```
This was after a bit of tinkering and it doesn't really work that great. This is sort of my initial caveman approach to it all. What I'd like to do next is replace the addition assignment with a subtraction assignment to see if that improves anything:
```
let x = 300 * noise(frameCount * 0.002)
let y = 500 * noise(frameCount * -0.006)
y -= random(3,2);
```
This KIND of works. It's just really not pretty at all, and I do not think this is ultimately going to be the correct solution.
Step 3 - READ MORE
I decided to open the p5.js Reference to find out more about noise() and discovered that you can offset the x and y variables of perlin noise generation. I'd like to try that in order to give our iceberg more of a northward bias. Here's a few snippets of the code that I am working on:
```
let Xoffset = 500;
let Yoffset = 1000;
let x, y;
x = width * noise(Xoffset);
y = height * noise(Yoffset);
y -= 2;
```
Multiplying both width and height by their noise offsets is aiding in getting smooth noise generation while also maintaining that y-bias I declared in the first few lines of the sketch. I am going to continue tinkering with the variables I've laid out and once I'm satisfied, I will upload my iceberg png and add a little color to my sketch.
Step 4 - Biased Randomness
I was able to successfully bias the perlin noise generation and now I'm left wondering if these numbers are truly random or not. Anyway, here is my code:
https://editor.p5js.org/flemin/sketches/TArpA0kMp
```
let Xoff = 0, Yoff = 1500;
let x, y;
let img;
function preload() {
img = loadImage("assets/iceberg.png");
}
function setup() {
createCanvas(400, 400);
x = width / 2;
y = height / 2;
}
function draw() {
background("#468df4");
x = width * noise(Xoff);
y = height * noise(Yoff);
y -= 5;
Xoff += 0.001;
Yoff += 0.003;
image(img, x, y, 30, 30);
}
```
It won't work without the iceberg png but luckily, it is attached here in this devlog.
Get Drawing, Moving and Seeing with Code - Spring 2025
Drawing, Moving and Seeing with Code - Spring 2025
Jack Fleming's work for class
More posts
- DMSWC - Final Megalog - JLyricgen32 days ago
- Week 11 - Squirrel Eat Squirrel World41 days ago
- Week 10 - Livecoding46 days ago
- Week 9 - Hydra and P5Live67 days ago
- Week 7 - Ecosystem Project [CONTINUED]82 days ago
- Week 6 - Ecosystem [DRAFT]88 days ago
- Week 5 - Smart Robot ALFIE95 days ago
- Week 4 - Generative Daily ProjectFeb 25, 2025
- Week 3 - Creature ClassFeb 17, 2025
Comments
Log in with itch.io to leave a comment.
Thanks for this step by step description. Nice approach to figuring out the northerly approach of the iceberg, trying things out, doing some research, and trying again. It’s a good way to try to figure out how to combine these two different ‘random’ noise generation techniques.
You may have to turn on markdown mode for your code in these devlogs!
Thanks for your feedback! It was a fun assignment to work on. Definitely going to try to get markdown mode on, just need to find where it is specifically in the settings, haha.