Week 3 - Creature Class
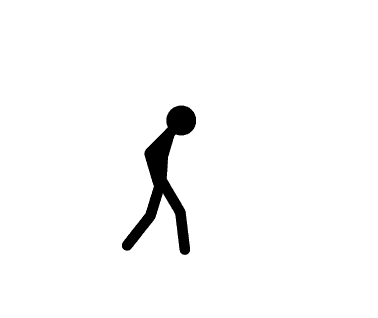
This week, we were tasked with creating a class for a ‘creature’ of our choosing to get better acquainted with object and class relationships in p5js. My idea for this assignment is to make a little interactive piece wherein an animated stick man will be walking freely around the canvas up until the moment you hover over it. It will be then that he chases your cursor around the canvas. I have nothing particular planned for a lose state to this sketch. If that is something you’d like to see, I can always add it in the future.
Step 1 - REVIEW
I unfortunately closed out of the tab while writing this devlog originally so some details from this step may be lost. Essentially, what I had done is take my code from class and change the ellipse to my stick man gif of choice. Additionally, I threw in some boundary detection so that the image would never travel fully off of the screen. Apart from that, the original class code had still been preserved. Here’s what that sketch looked like:
While it was not bad at this stage, I wanted to get rid of the text and make the background white so that the gif blended in seamlessly.
Step 2 - AESTHETICS
Aesthetics are an important part of this assignment, and I want this sketch to not look horrible while perhaps seeming intuitively interactive (even though it is going to be really simple). Therefore, I began by making the changes I discussed previously in the last step. Then, I used scale(); to flip the image horizontally when it changes direction. Here’s what that code looks like:
if (this.xchange < 0) {
scale(-1, 1);
image(gif, -this.x - 110, this.y, 110, 110);
scale(1, 1);
} else {
image(gif, this.x, this.y, 110, 110);
}
Step 3 - CHASE
Ok, so this is all looking and running good so far, though I need a bit more. I need to write the bit where the stick man will chase down our cursor when we hover over the canvas. I think to also increase our aesthetic value a bit, I’m going to change the cursor into an image itself. I would like to add more stick men on the screen, however, I did not download a transparent gif so each image would ultimately be overlapping another.
My first attempt isn’t necessarily bad, however, it does not behave how I would like it to since all of my if statements now contradict each other. Here’s what I mean:
if (this.x < mouseX) {
this.x++
}
if (this.x > mouseX) {
this.x--
}
if (this.y < mouseY) {
this.y++
}
if (this.y > mouseY) {
this.y--
}
This code being underneath my previous if statements which dictate the gif’s movement essentially overrides the original randomized movement. Therefore, this will not work for my project.
Step 3 - METHOD MAN
Alright, so I need a new method in my code (I found some helpful references on p5–I would have had no other way of knowing how to do this). First we begin with a new variable to check if the mouse’s X and Y coordinates are in the canvas. That looks like this:
let mouseOverCanvas = mouseX >= 0 && mouseX <= width && mouseY >= 0 && mouseY <= height;
and it goes into the draw function. I will also need to slightly alter the draw function with an if statement so the program can differentiate between when the cursor is on the canvas (stick man chases you) and when it is not (stick man wanders around). Here’s this code all together:
function draw() {
background(255);
let mouseOverCanvas = mouseX >= 0 && mouseX <= width && mouseY >= 0 && mouseY <= height;
if (mouseOverCanvas) {
stickMan.chaseMouse();
} else {
stickMan.move();
}
stickMan.display();
}
‘chaseMouse’ is my new method. This specifically is where things get complicated beyond my current java understanding and what we have done in class so far. The ‘atan2();’ function is used with mouse tracking in p5 a lot. As per what the reference says, it is “…most often used for orienting geometry to the mouse’s position, as in atan2(mouseY, mouseX). The first parameter is the point’s y-coordinate and the second parameter is its x-coordinate.” To be completely transparent, I ran out of places to look for help and asked ChatGPT how to incorporate it into my code. Here is what it spit out using the new method name:
chaseMouse() {
// Move towards the mouse
let dx = mouseX - this.x;
let dy = mouseY - this.y;
// Calculate angle to mouse
let angle = atan2(dy, dx);
// Move in the direction of the mouse
this.x += cos(angle) * 2; // Adjust speed by changing multiplier (2)
this.y += sin(angle) * 2;
}
I’m glad there are notes about what everything does in the code, and I asked it to explain everything to me so I can get a better grasp of atan2, especially with how its used to move objects in relation to whatever the “angle calculation” is. I am also super glad that it works! Altogether, my code is looking great now. The last and final change I’d like to make is to change the cursor to a money bag so the stick man has some incentive to chase it around the screen.
Step 4 - MONEY
https://editor.p5js.org/flemin/sketches/zNislHtbk
And there we have it! The final version of this creature class sketch. Hope you enjoyed!
Get Drawing, Moving and Seeing with Code - Spring 2025
Drawing, Moving and Seeing with Code - Spring 2025
Jack Fleming's work for class
More posts
- DMSWC - Final Megalog - JLyricgen32 days ago
- Week 11 - Squirrel Eat Squirrel World41 days ago
- Week 10 - Livecoding47 days ago
- Week 9 - Hydra and P5Live67 days ago
- Week 7 - Ecosystem Project [CONTINUED]82 days ago
- Week 6 - Ecosystem [DRAFT]88 days ago
- Week 5 - Smart Robot ALFIE95 days ago
- Week 4 - Generative Daily ProjectFeb 25, 2025
- Week 2 - Iceberg SimulationFeb 10, 2025
Comments
Log in with itch.io to leave a comment.
Perhaps you could find a stickman animation with a transparent background so the money is not obscured by the background of the gif. Also, maybe there could be some kind of feature for when the stickman does reach the money. Otherwise cool following algorithm!